Mastering Software Debugging: Techniques and Insights
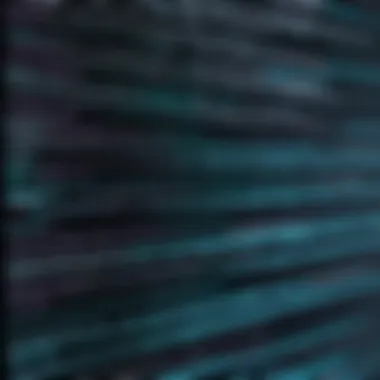
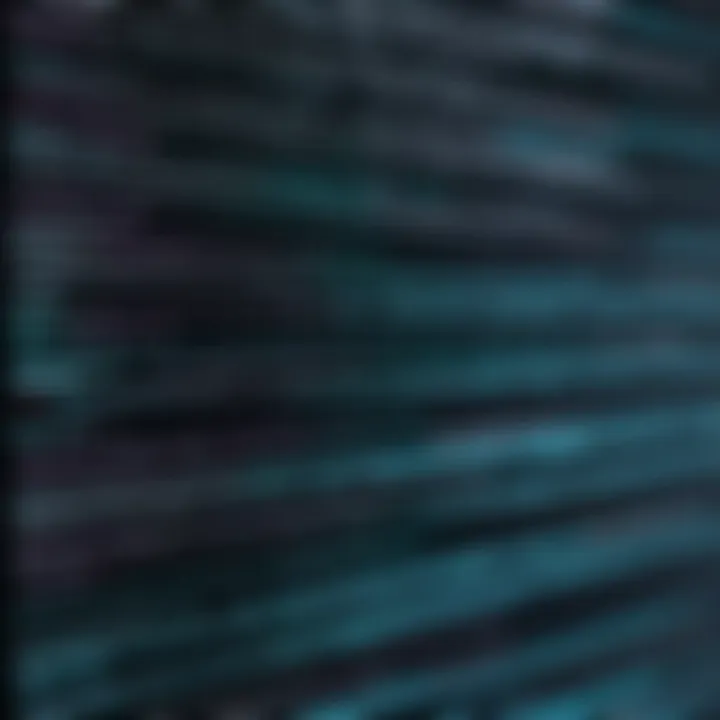
Intro
In today’s fast-paced technology landscape, software debugging is a fundamental aspect of software development. It is more than just identifying and fixing bugs; it is an intricate process that requires a deep understanding of the code and the systems involved. This article aims to explore the multifaceted world of software debugging, shedding light on its significance, methods, and best practices.
Understanding the Importance of Debugging
Debugging serves as a crucial safety net in the software development lifecycle. It ensures that the software meets the desired quality standards before reaching end-users. Without thorough debugging, errors can propagate, leading to significant malfunctions or even catastrophic failures. Thus, mastering debugging techniques is essential for developing reliable applications.
Key Features
The debugging process is characterized by several key features that enhance its effectiveness:
- Error Identification: Efficiently pinpointing errors within complex codebases.
- Root Cause Analysis: Understanding the underlying causes of issues rather than merely addressing symptoms.
- Iterative Refinement: Continually improving code quality through cycles of testing and fixing.
Within these features lies the foundation for a proactive approach to software quality assurance.
Overview of Features
The essence of debugging lies in its structured methodologies. A systematic approach helps in managing the complexity of software systems. Tools like integrated development environments (IDEs) and debugging frameworks provide developers with the capabilities to trace bugs effectively. This structured environment facilitates a more productive workflow, allowing for faster identification of problems.
Unique Selling Points
What makes effective debugging unique is its potential to drastically reduce the time needed for software deployment. Developers who are adept in debugging can deliver higher quality software in shorter timeframes. Some unique selling points include:
- Advanced Toolsets: Usage of sophisticated debugging tools like Visual Studio and Eclipse.
- Community Support: Access to online forums such as Reddit or specific platforms, which provide help for complex debugging issues.
- Documentation: Comprehensive resources on debugging strategies available on Wikipedia and related platforms.
Performance Evaluation
Assessing the performance of debugging processes reveals much about their efficiency. The evaluation entails examining speed and responsiveness, alongside resource usage.
Speed and Responsiveness
A key factor in debugging is the speed at which issues are identified and resolved. Tools that offer real-time feedback or integrated debugging features can significantly enhance responsiveness. When debugging is swift, it allows developers to maintain a smooth workflow, avoiding bottlenecks in software delivery.
Resource Usage
Effective debugging should aim to use system resources efficiently. Resource-heavy debugging processes can slow down development and lead to frustration. Tools that become a burden rather than an asset can hinder productivity. Therefore, developers should prioritize lightweight tools that do not excessively consume memory or processing power, ensuring optimal performance in the development environment.
"Debugging is not just a process, it's an essential skill that determines the health of the software product."
Understanding Software Debugging
The act of software debugging is a crucial element in the field of software development. Understanding this process is not just beneficial; it is essential for those involved in creating and maintaining software. Debugging involves identifying, isolating, and correcting bugs or errors in the software code. It is the key to enhancing software quality and ensuring optimal performance.
When developers comprehend the intricacies of debugging, they improve their problem-solving skills. They acquire a mindset that sees issues not merely as setbacks but as opportunities for learning and refinement. In this sense, debugging fosters a culture of continuous improvement—a cornerstone in software development. Additionally, effective debugging saves time and cost in the long run, as it reduces the need for extensive post-release fixes, ensuring that products are delivered with fewer defects.
Definition of Debugging
Debugging refers to the systematic process of diagnosing and resolving issues in software programs or applications. This mechanism varies across programming environments and can encompass a range of activities, from reviewing code to running debugging tools. At its core, debugging is about understanding how a program operates versus how it is expected to operate.
In technical terms, debugging may include various approaches such as static analysis, which examines the code without executing it, or dynamic analysis, where the program is run in a controlled environment. Furthermore, debugging tools, such as GDB for C/C++ or debug in Visual Studio, help developers step through their code, inspect variables, and alter execution paths to identify problematic areas efficiently.
Importance of Debugging in Software Development
Debugging is fundamental in software development for several reasons. Firstly, it significantly impacts the user experience. Software that lacks rigorous debugging may exhibit bugs that can frustrate users or lead to a lack of trust in the product. This can harm a company’s reputation.
Secondly, debugging contributes to maintaining the software lifecycle. As software evolves, new functionalities may introduce unexpected issues. Developers must prioritize debugging practices throughout the development cycle to ensure reliable and effective software.
Thirdly, effective debugging reduces costs associated with software maintenance. Identifying and solving bugs early in the development stage mitigates potential complications that could arise post-deployment, which are often more challenging and expensive to resolve.
"Effective debugging not only eliminates bugs but also fosters an environment where quality software can thrive."
Engagement with debugging processes is a powerful asset for developers and can lead to more successful software projects overall, making it a topic worth investing time to understand.
The Software Development Lifecycle
Understanding the Software Development Lifecycle (SDLC) is crucial for effective software debugging. This structured process not only encompasses various phases of software development but also highlights the interplay between development and debugging activities. Each phase offers its own set of challenges and opportunities. Developers can gain valuable insights into potential bugs and issues right from the beginning.
Overview of the Development Phases
The Software Development Lifecycle consists of several phases, each integral to the creation of functional software. These phases include:
- Requirements Gathering: This initial phase involves collecting and understanding what stakeholders need from the software. Proper documentation during this phase can prevent future misunderstandings.
- Design: Here, developers create architectural blueprints that outline how the software's components will interact. Flaws in design can lead to significant issues later on.
- Implementation: In this phase, actual coding takes place. Effective debugging starts here, as it addresses the initial code errors that might arise.
- Testing: Rigorous testing helps discover bugs. This phase is paramount, as it is the last opportunity to find flaws before the product goes live.
- Deployment: The software is released to users. Even at this stage, developers might find new bugs when real-world use occurs.
- Maintenance: This ongoing phase addresses issues and incorporates updates. Debugging remains a continuous effort throughout maintenance.
Integration of Debugging within Each Phase
Debugging is not limited to a single phase; it should be embedded within every aspect of the SDLC. Here’s how it integrates:
- In the Requirements Gathering Phase: Developers should clarify requirements to avoid misinterpretations that lead to bugs. Engaging stakeholders ensures that expectations align accurately.
- During the Design Phase: By conducting design reviews, developers can identify potential flaws that might later translate into bugs. Prototyping and modeling can aid in this detection.
- In Implementation: As code is written, using integrated development environments helps identify syntax errors immediately. Implementing coding standards can also minimize the introduction of bugs.
- Testing: This phase is crucial for debugging. Various types of testing, like unit and integration testing, actively seek out bugs in the software.
- When Deploying: Organisations might use beta testing to catch emerging bugs that were not evident in earlier stages. Gathering feedback becomes vital.
- In Maintenance: Continuous monitoring of software performance can reveal bugs, allowing for timely fixes.
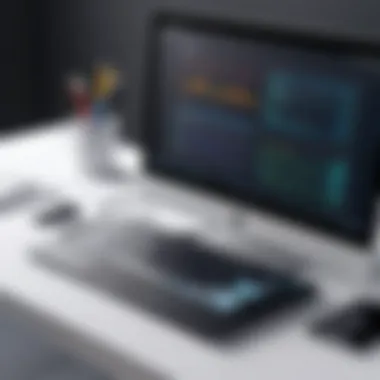
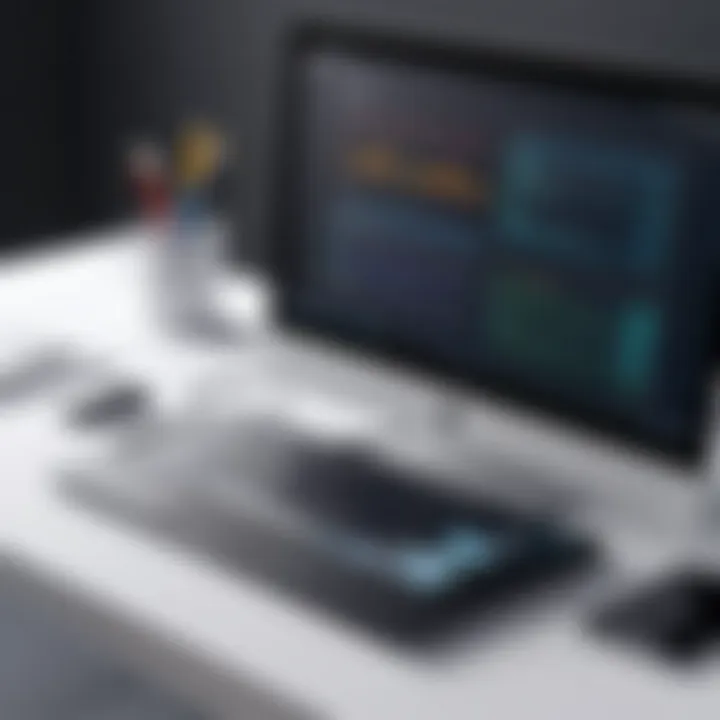
Debugging is a continuous process rather than a one-time event.
In summary, integrating debugging into every phase of the SDLC ensures that software is built with quality and reliability in mind. As software evolves, so does the need for ongoing debugging efforts, making it an indispensable aspect of the development lifecycle.
Common Types of Software Bugs
Understanding the different types of software bugs is essential for effective debugging. Identifying these bugs allows developers to craft appropriate solutions and maintain software quality. This section will focus on three main types of software bugs: syntax errors, logical errors, and runtime errors. Each type presents different challenges and requires distinct approaches for resolution.
Syntax Errors
Syntax errors occur when the code violates the grammatical rules defined by the programming language. These bugs are typically the easiest to identify since they prevent the code from compiling or running.
Common characteristics of syntax errors include:
- Misspelled Keywords: A simple typo can lead to a syntax error. For example, writing instead of in Python will result in an error.
- Incorrect Structure: Failing to follow correct formatting rules such as missing parentheses or braces.
- Mismatched Quotes: Not closing a string properly with the same type of quotation marks can cause issues.
To resolve syntax errors, developers can use Integrated Development Environments (IDEs) that highlight issues as the code is written. Debugging tools often provide hints or suggestions for correcting syntax issues, improving coding efficiency.
Logical Errors
Logical errors are more subtle than syntax errors. They occur when the code compiles and runs, but it produces incorrect or unexpected results. These bugs can be challenging to detect because the program appears to function properly, albeit incorrectly.
Some examples of logical errors include:
- Incorrect Calculations: An error in the mathematical operation can yield wrong results. For instance, using instead of in a division scenario.
- Flow Control Issues: An error in the logic of conditional statements may lead to desired paths not being executed.
- Infinite Loops: Failing to meet exit conditions can cause the program to run indefinitely.
To address logical errors, developers typically employ a methodical approach, often involving unit tests to isolate different parts of the code for validation. Review and debugging sessions can help trace the logic to find where things fail.
Runtime Errors
Runtime errors are bugs that occur while the program is executing. They are related to improper operations and can lead to crashes or abnormal termination of the software. Common examples include:
- Null Pointer Exceptions: Attempting to access or modify an object that is null. This is prevalent in languages like Java or C++.
- Array Index Out of Bounds: Accessing an element outside the range of an array can cause this type of error.
- Resource Management Issues: Failing to properly allocate or deallocate resources may lead to memory leaks or crashes.
Detecting runtime errors often requires dynamic analysis or debugging tools that monitor the execution of applications as they run. Analyzing logs and stack traces is crucial for diagnosing these errors.
By understanding the common types of software bugs, developers can implement more effective debugging strategies, enhancing overall code quality and performance.
Debugging Methodologies
In the realm of software engineering, debugging methodologies play a crucial role in ensuring high-quality products. A methodical approach to debugging can significantly reduce the time it takes to identify and resolve issues. Different methodologies provide distinct frameworks for handling bugs, depending on the context of the development process. By understanding these methodologies, developers can choose the most suitable approaches for their specific projects and applications.
Test-Driven Development
Test-Driven Development (TDD) is a practice where tests are written before the code itself. This approach emphasizes a cycle of writing a test, running it to see it fail, writing the minimum code necessary, and running the test again to ensure it passes.
Benefits of TDD include:
- Immediate Feedback: Developers receive quick confirmation of whether new code meets the intended design.
- Higher Code Quality: As tests are created first, the resulting code is often cleaner and more modular.
- Reduced Debugging Time: Bugs are identified shortly after they are introduced, leading to faster resolutions.
However, TDD requires discipline from developers. It may be challenging to implement in legacy codebases where writing tests first is not feasible. Additionally, TDD can initially slow down the development pace, as creating tests for each new feature is necessary.
Behavior-Driven Development
Behavior-Driven Development (BDD) extends the principles of TDD by focusing on the behavior of an application from the end-user’s perspective. BDD encourages collaboration among developers, testers, and non-technical stakeholders to define expectations through clear, structured scenarios.
Some key characteristics of BDD include:
- Collaboration: BDD fosters communication among all parties, improving the final product.
- User-Focused: It emphasizes the application's behavior, ensuring that it meets user needs.
- Executable Specifications: BDD scenarios can often be executed against the code, serving as both documentation and tests.
Challenges may arise in alignment and understanding among team members. Moreover, the initial setup for BDD can be complex, as it requires buying into a collaborative culture.
Exploratory Testing
Exploratory Testing is a non-linear approach to testing where testers explore the application freely without predefined scripts. This methodology empowers testers to use their intuition, experience, and knowledge about the software to uncover issues that may not be discovered through traditional scripted testing.
The benefits of Exploratory Testing include:
- Uncovering Hidden Bugs: Testers can identify unexpected issues that scripted tests may overlook.
- Adaptability: It allows for the flexibility of testing aspects of the application that might change during development.
- Rapid Feedback Loop: This method can quickly gather critical insights about the software, speeding up the debugging process.
Nonetheless, Exploratory Testing requires skilled testers who can think critically and creatively. It may not provide the coverage that scripted testing does, but its value lies in discovering nuanced issues that enhance the overall quality of the software.
Understanding and implementing different debugging methodologies can profoundly impact the efficiency and effectiveness of software development. Each methodology offers unique benefits and considerations that developers should weigh to improve their debugging processes.
Tools for Software Debugging
In the realm of software development, the right tools can enhance efficiency and accuracy in the debugging process. Tools for software debugging serve not only to identify issues but also to provide insights that can lead to better coding practices and improved software reliability. These tools vary in nature, each designed to address specific aspects of the debugging phase. This section delves into the most prominent tools used by developers today, emphasizing their unique benefits and considerations.
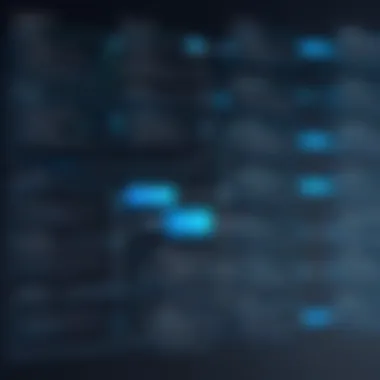
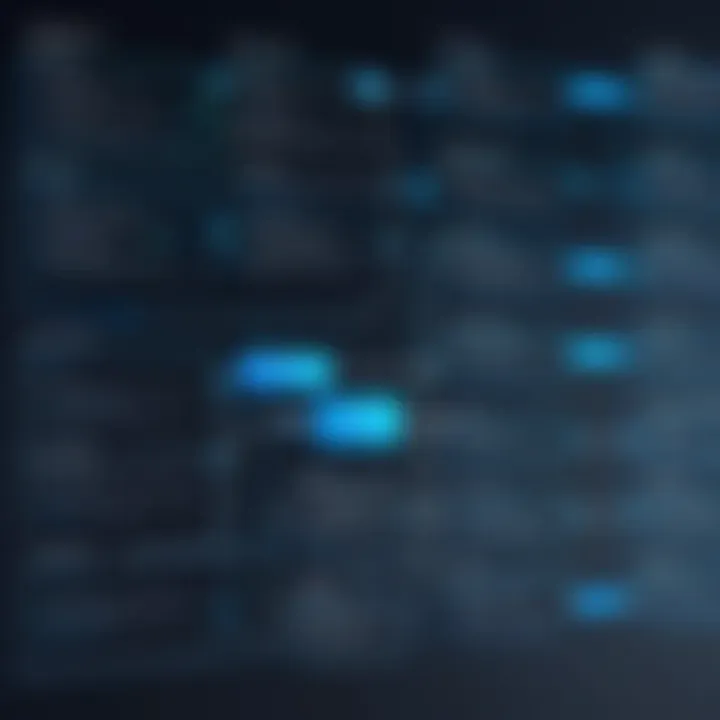
Integrated Development Environments (IDEs)
Integrated Development Environments, commonly known as IDEs, play a pivotal role in modern software development. They combine different tools in one interface, enabling developers to write, compile, and debug their code efficiently. Popular IDEs such as Visual Studio, IntelliJ IDEA, and Eclipse offer built-in debugging capabilities that streamline the process of locating and fixing bugs.
An IDE facilitates real-time code analysis, which can significantly reduce the time spent debugging. Features like breakpoints, stack traces, and variable watches provide developers with clear visibility into their code's execution flow. This visual representation of how the code operates is invaluable when attempting to pinpoint the source of a problem. Moreover, IDEs support various programming languages, making them versatile tools for a wide range of projects.
Using an IDE can improve overall productivity by integrating source control, project management, and task tracking within a single environment. However, one must consider the learning curve associated with complex IDEs, which can initially overwhelm new developers.
Static Analysis Tools
Static analysis tools analyze code without executing it, allowing developers to detect potential errors and coding standard violations before runtime. These tools, such as SonarQube, ESLint, or Checkstyle, provide crucial feedback on code quality, which aids in maintaining a clean codebase.
The primary advantage of static analysis is its ability to identify issues early in the development lifecycle. This proactive approach can lead to significant cost savings, as fixing bugs at this stage is generally less expensive than addressing them after deployment. Furthermore, static analysis tools can enforce coding standards across a team, ensuring that all members follow best practices consistently.
Nevertheless, these tools are not foolproof. They can produce false positives, especially in complex codebases, which may lead to unnecessary alarms. Developers should prioritize their findings carefully and balance automated checks with manual review processes.
Dynamic Analysis Tools
Dynamic analysis tools operate by executing the program during runtime. They monitor the performance of code in real-time, which helps identify issues like memory leaks, race conditions, and performance bottlenecks. Tools like Valgrind and Dynatrace provide insights that are critical when assessing the runtime behavior of applications.
The benefits of dynamic analysis lie in its ability to expose issues that are often missed during static analysis. For instance, performance profiling is essential for understanding how applications behave under various load conditions. Dynamic analysis tools can also assist in uncovering underlying issues related to resource allocation and usage.
However, dynamic analysis tools often require a more extensive setup and can be resource-intensive. Their effectiveness relies heavily on the quality of the test cases used during execution. Without comprehensive testing scenarios, the insights gained may not accurately reflect potential issues in the software.
Ultimately, the choice of tools depends on various factors including the project's complexity, language, and team preferences. Using a combination of these tools can provide a more holistic view of the software's health, significantly improving the debugging process.
Best Practices for Effective Debugging
Debugging is a crucial part of software development. Following best practices can significantly enhance the debugging process. These practices help software developers identify and fix issues more efficiently. This section covers three fundamental best practices: reproducing bugs, utilizing version control, and keeping a bug log.
Reproducing Bugs
Reproducing bugs is essential for any debugging effort. When developers can recreate a bug, they gain insights into its behavior. This understanding can lead to an effective solution. A systematic approach to reproducing bugs is beneficial. Start by gathering information about the bug, including error messages, system states, and user actions.
- Document Steps: Write clear instructions on how to reproduce the bug.
- Use Consistent Environment: Ensure the software runs in the same environment where the bug occurred. Changes in hardware or software can affect the outcome.
- Examine Input Values: Different inputs can lead to different behaviors. Be thorough.
Reproducing a bug several times can confirm it exists and help to clarify its root cause. Consistent reproduction is key to ensuring that any eventual fix addresses the underlying problem.
Utilizing Version Control
Version control systems are invaluable tools in the debugging process. They allow developers to track all changes made to the codebase. This capability aids in identifying when bugs were introduced. Utilizing version control can offer several significant advantages:
- Rollback Capabilities: If a new change introduces a bug, reverting to a previous version may quickly resolve the issue.
- Branching and Merging: Developers can create branches for new features without affecting the main codebase. This isolation can clarify whether a change causes a bug.
- Collaboration: Teams can work on different aspects of a project without overwriting each other's changes, reducing the likelihood of bugs due to conflicting code.
Effective use of version control not only simplifies debugging but also enhances collaboration within development teams.
Keeping a Bug Log
A bug log serves as an essential tool for tracking issues throughout the software development process. This log helps provide clear records of existing issues, solution attempts, and progress over time. A detailed bug log may include:
- Identification Number: Assign a unique ID for easy reference.
- Description: A brief note outlining the nature of the bug.
- Status: Keep track of whether the bug is open, in progress, or resolved.
- Steps to Reproduce: Include the steps necessary to recreate the issue.
- Resolution: Note how the issue was finally resolved, if applicable.
Keeping a bug log improves organization within a team and enhances accountability. Tracking issues with this method allows developers to prioritize effectively and address time-sensitive problems efficiently.
By adhering to these best practices, developers can streamline their debugging efforts, ultimately leading to more robust software.
Common Challenges in Debugging
Debugging is a vital part of software development, but it is not without its inherent challenges. Understanding these obstacles is crucial for software developers, IT professionals, and students in tech fields. Addressing these challenges can lead to more effective debugging strategies and ultimately produce better software.
Complex Software Systems
One of the primary challenges in debugging is dealing with complex software systems. Modern applications often consist of numerous components, libraries, and frameworks. Each part can interact in unexpected ways. This complexity makes it difficult to isolate bugs. When a problem arises, identifying which particular segment is causing the issue becomes a daunting task. Furthermore, the interconnected nature can hide bugs that might not show their effects until numerous steps or other parts of the system are involved.
Developers must often navigate through various dependencies. This examination can be frustrating when changes in one area inadvertently affect another, leading to a ripple effect of bugs. Adequate testing and modular design practices can mitigate some of these issues. However, even the most robust systems may face hidden complexities. Therefore, developers should adopt a methodical approach to dissect and analyze each component during the debugging process.
Limited Documentation
Limited or poor documentation is another significant barrier to effective debugging. Documentation serves as a guide for developers to understand how the software is supposed to function. When documentation is lacking, outdated, or unclear, it becomes increasingly challenging to identify the source of an issue. This is particularly true in large codebases where the original authors may no longer be available to clarify intent or functionality.
In these scenarios, developers may have to rely on their assumptions or spend time reverse-engineering the code to understand its structure and functionality. This process can be time-consuming and lead to frustration. To combat this challenge, maintaining comprehensive and up-to-date documentation is essential. Additionally, using comments effectively within the code can provide immediate context and assist those who work on the project in the future.
Time Constraints
Time constraints represent a critical challenge in the debugging process. In the fast-paced environment of software development, pressure to deliver products quickly can lead to rushed debugging efforts. When timelines are tight, developers might prioritize getting features live over ensuring the software is free of bugs. This tendency can result in longer-term technical debt, creating more issues down the line.
Moreover, under time constraints, developers may not have the luxury to perform thorough testing or take the time to experiment with potential solutions. Instead, they often rely on quick fixes that may not address the root of a problem.
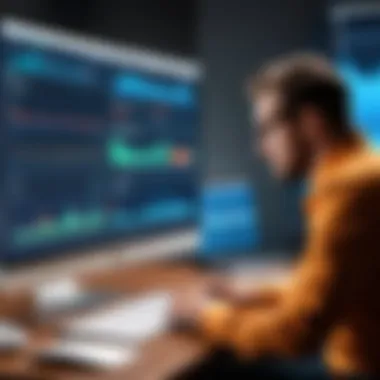
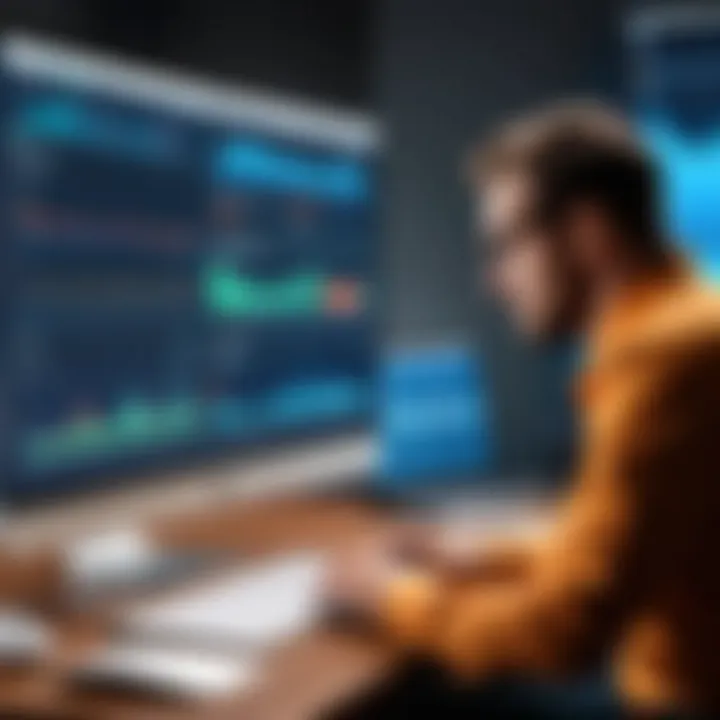
To address this challenge, it's important to allocate adequate time for debugging within project schedules. Planning for debugging as part of the software development lifecycle can help ensure that developers have the necessary resources to address bugs properly, thereby maintaining software quality without sacrificing speed.
The efficiency of debugging practices significantly impacts overall software reliability. Addressing challenges head-on can lead to more robust solutions and a more reliable product.
By being aware of these common challenges—complex software systems, limited documentation, and time constraints—developers can adopt more effective debugging strategies. This proactive approach ultimately contributes to higher software quality and a smoother development process.
Advanced Debugging Techniques
Understanding advanced debugging techniques is crucial for software developers and IT professionals striving to create high-quality applications. These techniques help identify and resolve complex issues that may arise during the software lifecycle. Advanced debugging techniques not only enhance the efficiency of the debugging process but also contribute to a deeper understanding of application performance. Employing these methods ensures that potential problems are addressed swiftly, resulting in more reliable and maintainable software.
Automated Debugging Tools
Automated debugging tools represent a significant advancement in software development. These tools can quickly identify bugs that would take much longer to discover manually. They automate the process of checking the code for common issues, which saves time and allows developers to focus on more critical tasks. Tools like SonarQube and Sentry analyze code for vulnerabilities, helping developers maintain code quality.
Benefits of automated debugging tools include:
- Time Efficiency: Automated tools can scan large codebases faster than manual reviews.
- Consistency: These tools consistently apply the same set of rules and checks, reducing human error.
- Actionable Insights: They provide concrete suggestions on how to fix specific issues, streamlining the debugging process.
However, it's essential to consider that while automated tools are helpful, they should not replace manual debugging entirely. Human intuition and experience play vital roles in understanding complex issues that tools may not detect.
Memory Leak Detection
Memory leaks pose a significant challenge in software development, often leading to performance degradation over time. Detecting and resolving memory leaks is essential for ensuring that applications run optimally. Tools designed for memory leak detection, such as Valgrind and LeakSanitizer, help developers identify areas where memory is allocated but not released properly.
Key aspects of memory leak detection include:
- Identification: Using memory profiling tools to pinpoint the sources of leaks can reduce debugging time.
- Resolution: Addressing leaks not only enhances performance but also increases the stability of software.
- Preventive Measures: Developers should adopt best practices in memory management to avoid leaks altogether.
Incorporating memory leak detection early in the development process can result in a more robust application.
Performance Profiling
Performance profiling is another advanced debugging technique that focuses on identifying bottlenecks within applications. Profiling tools provide insights into how different parts of the application consume resources and how this affects overall performance. Tools like New Relic and AppDynamics are commonly used to assess application performance.
Key benefits of performance profiling include:
- Resource Allocation Insights: Profiling helps understand how memory, CPU, and other resources are utilized.
- Bottleneck Identification: Developers can pinpoint performance bottlenecks and take corrective action.
- Optimized Performance: By analyzing performance data, software can be optimized, resulting in a better user experience.
Using performance profiling tools in combination with other debugging techniques can lead to significant improvements in application performance and reliability.
Future Trends in Debugging
The realm of software debugging continues to evolve. As developers’ needs grow more complex, the tools and techniques for debugging must keep pace. This section will explore how emerging trends such as artificial intelligence and enhanced frameworks are shaping the future of debugging. Understanding these trends can offer insights into not only improving bug detection but also streamlining overall software quality assurance.
AI and Machine Learning in Debugging
Artificial intelligence (AI) and machine learning (ML) are becoming increasingly relevant in debugging software. These technologies can analyze vast amounts of data more efficiently than human developers. By utilizing AI techniques, tools can learn from previous bugs and identify patterns that may not be immediately obvious.
Several benefits arise from applying AI in debugging:
- Enhanced Bug Detection: AI algorithms can recognize unusual behaviors in software. This proactivity minimizes the time taken to discover an issue.
- Predictive Analysis: AI can predict potential future bugs based on historical data. This anticipation empowers developers to address issues before they cause significant problems.
- Automated Suggestions: Machine learning models can suggest fixes for detected bugs. This not only saves time but also helps developers to implement changes efficiently.
However, integrating AI in debugging also presents challenges. Developers need to ensure data quality. Otherwise, poor data will lead to inaccurate predictions. Additionally, the cost and complexity of implementing AI solutions can be prohibitive for some teams.
"The future of software development is heavily reliant on AI-driven processes. Debugging will not remain untouched by this revolution."
Improved Debugging Frameworks
As the demand for robust software solutions grows, the frameworks used for debugging must also advance. Improved debugging frameworks are vital as they can make the debugging process more efficient and user-friendly.
Key aspects of enhanced debugging frameworks include:
- Integration Capabilities: Modern frameworks should seamlessly integrate with various development tools and environments. This ensures that developers can work in their preferred ecosystems without sacrificing debugging efficiency.
- Real-time Collaboration: In a connected world, debugging frameworks with collaborative features promote teamwork. Developers can share insights and findings effortlessly, reducing the time to resolve issues collectively.
- User-Friendly Interfaces: Complexity can hinder the debugging process. Improved frameworks focus on creating intuitive interfaces that guide developers through the debugging steps clearly.
- Support for Multiple Languages: As software becomes increasingly polyglot, frameworks must support debugging across different programming languages. This capability helps developers work more flexibly.
Adaptation to these new frameworks demands continuous learning. Developers must familiarize themselves with features to leverage them fully. Keeping an eye on advancements is crucial as the landscape of software development is rapidly changing.
Closure
In the realm of software development, debugging holds a position of considerable significance. This article has explored the various facets of debugging, emphasizing its role as a necessary process to ensure the quality and functionality of software applications. Without debugging, the risk of undiscovered bugs increases, potentially leading to costly errors and user dissatisfaction.
The Ongoing Importance of Debugging
Debugging is not just a task; it is an ongoing commitment to maintaining the integrity of software products. Continuous integration and deployment practices require that debugging becomes part of everyday routine for developers. Debugging ensures that newly added code does not introduce new issues, which can compromise previously established functions.
Effective debugging practices lead to a more reliable software experience for users.
"Debugging is like being the detective in a crime movie where you are also the murderer."
It challenges developers to think critically about their work and fosters a culture of quality. Moreover, incorporating advanced techniques such as automated tools and analytics can streamline the debugging process, enabling quick resolutions to complex problems.
Additionally, as technology evolves, the importance of debugging cannot be overstated. As software systems become increasingly intricate, the methodologies that govern debugging must also advance. This evolution will ensure that professionals can manage the new challenges that arise with each advancement in technology. By investing time and resources into refining debugging practices, organizations can not only elevate their software quality but also enhance user trust and satisfaction.
In summary, the consistent focus on debugging is a vital component in the overall success of software development projects. It not only mitigates risks of significant failures but also encourages a proactive approach to software performance and quality assurance.