Exploring JavaScript Libraries and Frameworks: A Deep Dive
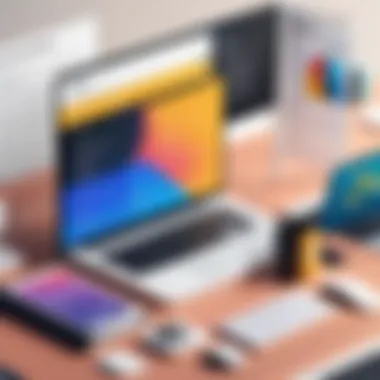
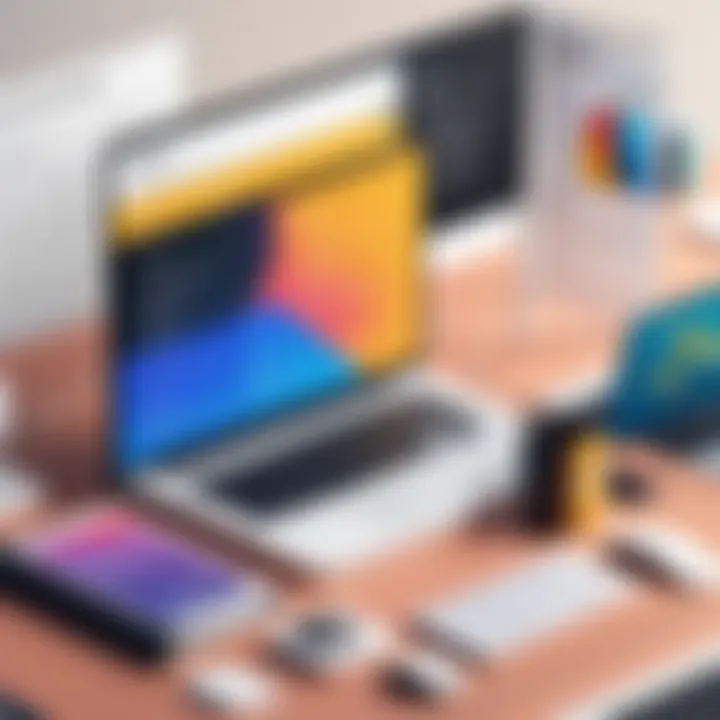
Intro
JavaScript stands as a cornerstone of web development. With its evolution, it spurred the creation of libraries and frameworks that simplify and enhance the development process. Understanding these tools is crucial for software developers, IT professionals, and students alike. This article aims to dissect these technologies, focusing on their features, performance, and use cases. The discussion will provide valuable insights that align with current trends and user needs.
Key Features
Overview of Features
JavaScript libraries and frameworks offer a plethora of features that enable developers to create interactive and efficient web applications. Libraries, such as jQuery and Lodash, often provide specific functionalities that can be integrated into projects with ease. They focus on code reuse and modularity, allowing developers to implement features without starting from scratch.
On the other hand, frameworks like Angular and React offer comprehensive solutions for building applications. They present a structured environment with guidelines and best practices, promoting a coherent codebase. Frameworks encapsulate a wide range of functionalities, including state management, routing, and templating, streamlining complex development tasks.
Unique Selling Points
The unique selling points of these tools stem from their design philosophy and intended purposes.
- Libraries are flexible and less prescriptive, empowering developers to choose when and how to use the code.
- Frameworks enforce architectural patterns, leading to better team collaboration and maintainable code over time.
According to their specific needs, a developer or a team can select either option, weighing flexibility against structure. This adaptability is critical in meeting the diverse demands of modern web applications.
Performance Evaluation
Speed and Responsiveness
Performance stands as a key criterion for evaluating JavaScript libraries and frameworks. Many libraries provide quick integration for specific tasks, which can enhance the speed of development. However, performance isn't solely about speed in executing code; it also includes responsiveness in user interactions. Success relies on minimizing lag in UI updates, especially in single-page applications.
Frameworks, like React, optimize rendering processes. They utilize virtual DOM techniques to efficiently update portions of the UI, ensuring that applications remain responsive under load. This performance evaluation is critical when selecting a tool, especially for applications with heavy user interaction.
Resource Usage
Another vital attribute is resource usage, including memory and bandwidth consumption. Lightweight libraries tend to have lower overhead, making them ideal for smaller applications or when rapid prototyping is required. However, larger frameworks often provide extensive features that, while powerful, may consume more resources.
Understanding the resource demands of each option helps developers make informed choices when designing scalable solutions that meet user expectations.
"Choosing the right tool—a library or a framework—depends not only on the project requirements but also on the desired performance outcomes."
Prelude to JavaScript Libraries and Frameworks
JavaScript libraries and frameworks play a crucial role in web development today. They not only simplify the programming process but also enhance the capabilities of web applications. By using these tools, developers can save time and effort. This section introduces the essence of these technologies and their significance in the modern coding landscape.
Defining JavaScript Libraries
A JavaScript library is a collection of pre-written JavaScript code that facilitates common tasks. Developers use libraries to avoid redundancies and streamline code development. jQuery is a prime example, allowing for efficient DOM manipulation and event handling with minimal code. Libraries focus primarily on providing specific functionalities rather than imposing a structure on the codebase. This allows developers to integrate them into existing projects flexibly.
Using a library means you choose which functions to use, maintaining greater control over their workflows. Libraries enhance productivity by condensing large processes into simpler commands. Therefore, understanding libraries provides insight into achieving efficiency in development.
Understanding JavaScript Frameworks
Contrary to libraries, a JavaScript framework is a more structured approach to coding. It lays out a skeleton for developers to build upon, establishing guidelines on how an application should be constructed. Angular is a well-known framework that offers a comprehensive solution for creating single-page applications (SPAs). Frameworks dictate the design pattern and often require a specific way of organizing code.
Adopting a framework can lead to a steeper learning curve. However, it also encourages consistency in projects, making it easier for teams to collaborate. The organizational structure aligns with modern practices, which can be beneficial in larger projects or complex applications. Understanding frameworks provides a roadmap for developers seeking to build robust web applications.
Key Differences Between Libraries and Frameworks
The distinction between libraries and frameworks is vital for developers. Here are key points that highlight their differences:
- Control vs Structure: Libraries offer a la carte options, allowing developers more control. Frameworks impose a design structure, reducing freedom but enhancing organization.
- Usage: Libraries are typically called upon when specific functionality is required. In contrast, frameworks are used as the foundational base from which developers build their applications.
- Learning Curve: Libraries are generally easier to pick up due to their focused functionalities. Frameworks, while more comprehensive, necessitate more time investment to understand their architecture.
Understanding these differences can significantly influence how a developer approaches a project, helping to choose the right tool for the task at hand.
In summary, recognizing the roles of libraries and frameworks in JavaScript is essential for modern web development. By leveraging these tools, developers can create sophisticated and efficient applications.
The Evolution of JavaScript: A Historical Context
The evolution of JavaScript is pivotal to understanding its current form and function. JavaScript has flourished into a versatile language, essential for web development. This transformation highlights numerous historical milestones that have not only shaped the language but also influenced who it serves today. By examining its evolution, one can appreciate the breadth and depth of its capabilities, as well as foresee future trends.
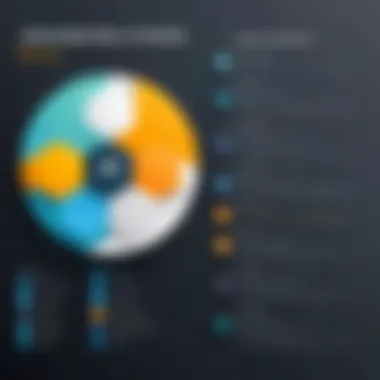
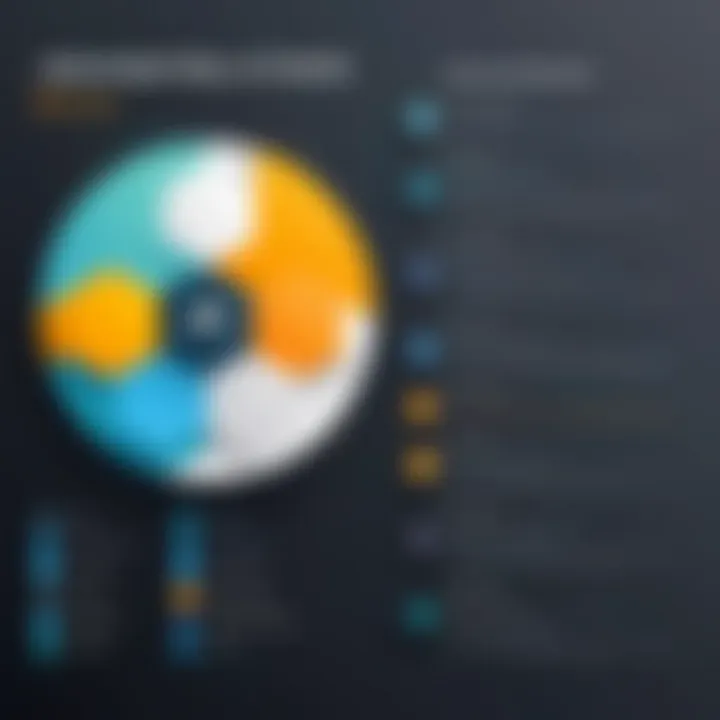
The Genesis of JavaScript
JavaScript was created in 1995 by Brendan Eich while he was working at Netscape Communications Corporation. Initially named Mocha, it quickly transformed to LiveScript, and ultimately settled on the name JavaScript to leverage the popularity of Java. This initial release aimed to add dynamic functionality to web pages, a breakthrough at the time. The simplicity of JavaScript allowed developers to enhance user interactions without complex processes.
In its early days, JavaScript's role was limited largely to form validation and simple animations. However, as the web grew more complex, developers began to demand more robust capabilities. This period saw the rise of frameworks and libraries that expanded the language's functionality significantly.
Major Milestones in JavaScript Development
Several milestones mark JavaScript’s development journey:
- ECMAScript Standardization (1997): JavaScript was standardized under the ECMA-262 specification. This was crucial for promoting consistency across different browsers. It allowed developers to rely on a common set of functionalities regardless of the platform.
- Introduction of AJAX (2005): The advent of Asynchronous JavaScript and XML (AJAX) revolutionized web applications. It enabled asynchronous data loading, allowing web pages to update without requiring a full reload. This resulted in smoother user experiences and led to the development of rich internet applications.
- Rise of JavaScript Frameworks (mid-2000s): Libraries like jQuery emerged, simplifying DOM manipulation and bridging gaps between different browsers. This was a turning point, leading to a growing ecosystem of libraries and frameworks.
- Node.js (2009): The introduction of Node.js marked a significant expansion of JavaScript's role beyond the browser. Developers could now use JavaScript for server-side applications. This opened new avenues for JavaScript, creating a unified approach to full-stack development.
- ES6 (2015): The sixth edition of ECMAScript introduced numerous advanced features such as arrow functions, classes, and modules. This update streamlined syntax and improved code efficiency, further establishing JavaScript as a modern programming language.
"The history of JavaScript is more than just a timeline; it's a testament to its adaptability and resilience in the face of evolving technology."
These milestones underscore a trajectory of continual growth and refinement. As JavaScript continues to evolve, understanding its past allows developers to leverage its full potential in modern applications. The history of JavaScript frames our current discourse on libraries and frameworks, emphasizing their increasingly sophisticated applications in web development.
Popular JavaScript Libraries
JavaScript libraries play a crucial role in modern web development. They provide pre-written JavaScript code that can be used to simplify tasks. This saves time and enhances productivity for developers. Focusing on popular libraries allows us to appreciate their specific features and the problems they solve for applications today.
jQuery: A Legacy in Simplifying DOM Manipulation
jQuery is one of the most widely used JavaScript libraries. It was created to enhance the way developers interact with HTML documents and how they manipulate the Document Object Model (DOM). With jQuery, tasks like animations, event handling, and AJAX are more straightforward. One significant advantage of jQuery is its cross-browser compatibility. This means code written with jQuery works consistently across different web browsers, reducing the need for extensive testing.
Developers appreciate jQuery for its succinct syntax. For example, a simple line of code can hide an HTML element, whereas it might require more lines in plain JavaScript. This reduces cognitive load and allows developers to focus on logic rather than the minutiae of JavaScript syntax. Yet, as web standards have evolved, some argue that jQuery's relevance may be decreasing due to native browser features that replicate its functionality. Still, many existing projects utilize jQuery, and it remains a valuable skill for web developers today.
D3.js: Data-Driven Document Manipulation
D3.js is a powerful JavaScript library focused on data visualization. It allows developers to bind data to the DOM and apply data-driven transformations to the document. D3.js supports a range of visual formats, from bar charts to complex interactive visualizations. Its significant strength lies in its ability to generate dynamic and interactive graphics that respond seamlessly to data changes.
The rich feature set of D3.js is best understood through practical examples. When visualizing data, a developer can create SVG graphics that are bound to real datasets. For instance, a live updating dashboard can be created where charts adjust automatically as new data comes in. Despite its steep learning curve, many developers find that mastering D3.js provides rewarding results, especially for projects centered on data representation.
Lodash: Utility Functions Made Easy
Lodash is a modern utility library that provides helpful functions for common programming tasks using JavaScript. Aimed at simplifying array and object manipulation, it offers developers a variety of functions including mapping, filtering, and deep cloning. Lodash enhances productivity by cleaning up repetitive tasks that developers frequently encounter.
One of Lodash's best features is its consistency across environments. This means it works uniformly in Node.js and browser contexts. The library’s modular design allows developers to import only the functions they need. This results in lighter code and improved performance. For instance, using from Lodash is typically clearer and more concise than the built-in . This clarity aids in written communication within the codebase, making it easier for teams to collaborate efficiently.
"Adopting libraries like Lodash can significantly cut down on development time while helping maintain code clarity and readability."
Prominent JavaScript Frameworks
JavaScript frameworks are essential tools that facilitate efficient and organized development for web applications. Understanding prominent frameworks is critical in modern web development as they offer structured guidance, reusable components, and a strong community backing. As developers face increasingly complex requirements, adopting a suitable framework can significantly streamline project execution and improve maintainability.
Angular: A Comprehensive Framework for Building SPAs
Angular is a platform built on TypeScript, crafted for creating single-page applications (SPAs). Developed by Google, this comprehensive framework encompasses a suite of tools that optimize the development process. Its two-way data binding feature enhances synchronization between the model and the view, minimizing the need for extensive coding. Moreover, Angular promotes component-based architecture, which results in modular design and reusable components. This is crucial for large applications, ensuring that teams can work collaboratively without code collisions.
One prominent aspect of Angular is its dependency injection system, which simplifies the management of services and enhances the testability of applications. Furthermore, it incorporates robust routing capabilities, allowing developers to manage navigation and deep linking effortlessly.
React: A Library Focused on UI Components
React, developed by Facebook, stands out among JavaScript libraries due to its focused approach on building user interfaces. It facilitates the creation of dynamic, single-page applications with efficiency. The core of React is its component-based architecture, where each UI element is encapsulated as a component. This promotes reusability and a clear separation of concerns.
React uses a virtual DOM to optimize rendering processes, ensuring that only the parts of the UI that actually need updates are modified. This leads to significant enhancements in performance, especially in applications with complex state management. Moreover, React’s ecosystem offers tools like React Router and Redux for state management, which further enhance its capabilities for building large-scale applications.
"React allows developers to create interactive UIs effortlessly, focusing on the desired output rather than the underlying complexities of DOM manipulation."
Vue.js: The Progressive Framework
Vue.js offers a flexible and progressive framework that can be incrementally adopted. Unlike Angular or React, Vue.js is designed to be approachable, making it a suitable option for developers at any experience level. Its core library enables data binding and the composition of components, while the ecosystem encompasses additional resources for more complex needs.
One of the advantages of Vue.js is its size and simplicity. It integrates easily into projects and can be incrementally adopted, enabling developers to enhance existing applications without overwhelming changes. Vue’s reactivity system ensures that components respond dynamically to data changes, similar to React's methodology.
Vue’s community has grown significantly, leading to abundant resources and support. While it may not be as widely adopted as React or Angular, its ease of use and flexibility make it a strong competitor in the market.
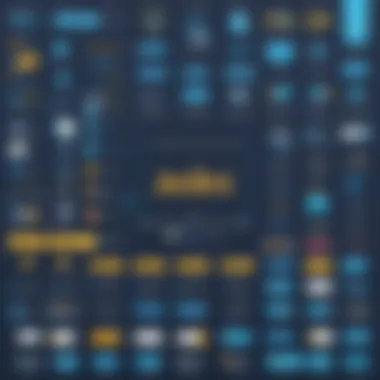
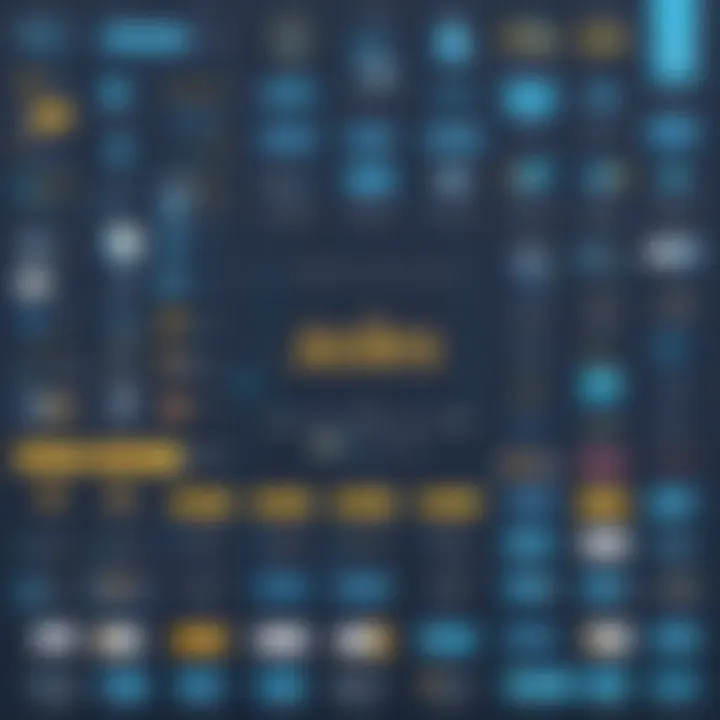
In summary, understanding these prominent JavaScript frameworks—Angular, React, and Vue.js—equips developers with necessary tools to tackle modern challenges in web application development. Each framework offers unique strengths and considerations, making careful selection essential for optimal results.
Advantages of Using JavaScript Libraries and Frameworks
JavaScript libraries and frameworks offer numerous advantages that can greatly enhance productivity and effectiveness in web development. In an era where speed, efficiency, and maintainability are paramount, understanding these advantages can inform choices that lead to successful software projects. Libraries and frameworks are not just coincidences in coding; they represent a significant shift in how developers approach building applications. The benefits these tools provide are manifold, including enhanced efficiency, code reusability, and robust community support.
Enhanced Efficiency in Development
Efficiency is one of the most compelling reasons to use JavaScript libraries and frameworks. With these tools, developers can leverage pre-written code and functionalities that save time during the development process. This allows them to focus on the unique aspects of their projects rather than reinventing the wheel. For instance, a library like jQuery simplifies DOM manipulation, making it easier to handle events and animations without extensive coding.
Moreover, frameworks like Angular and React come with built-in features like routing and state management, further streamlining development. When teams utilize these structures, they typically see a reduction in development time and a lower chance of errors, as the tested code is reused across applications. A well-established library or framework means fewer bugs and faster iterations. This is essential in a competitive landscape where time-to-market can define success.
Code Reusability and Modularity
Code reusability is intrinsic to the philosophy of using libraries and frameworks. By breaking functionality into small, manageable modules, developers can reuse code snippets across multiple projects, which contributes to reduced redundancy. This modularity not only minimizes the overall codebase but also simplifies maintenance. For example, Lodash provides utility functions that remain applicable across various contexts, making it easy to incorporate into different projects.
This approach facilitates better organization of code, allowing developers to create cleaner and more understandable structures. Eventually, this improves collaboration, as team members can grasp the layout of the codebase without needing extensive documentation.
Community Support and Ecosystem
Another significant advantage is the robust community support surrounding many JavaScript libraries and frameworks. Popular tools often have large communities of developers and contributors. This is essential for several reasons:
- Resources: Extensive documentation, tutorials, and forums provide valuable learning opportunities and assistance for developers at all skill levels.
- Updates: Frequent updates and improvements are driven by community contributions. This fosters an environment where libraries and frameworks evolve in response to developers’ needs.
- Plugins: Many libraries and frameworks support various plugins and extensions, allowing projects to expand features without heavy lifting.
In essence, an active community can significantly enhance the usability and longevity of a JavaScript library or framework. Developers can rely on collective knowledge and experience, leading to faster problem-solving and reduced friction in the development process.
Generally, leveraging JavaScript libraries and frameworks not only accelerates the development process but also improves code quality and project manageability.
Critiques of JavaScript Libraries and Frameworks
In the world of web development, JavaScript libraries and frameworks have become tenets of modern programming. However, their popularity does not come without critique. Evaluating these tools is essential for developers to understand their limitations, which can influence project success. The criticisms often touch on two key areas: heavy dependencies and performance issues, alongside the learning curve for new developers. Discussing these critiques helps professionals make informed decisions about what tools to adopt for their projects.
Heavy Dependencies and Performance Issues
Heavy reliance on libraries and frameworks can lead to several issues. One significant concern is performance. Using a large library may provide more functionality, but it also means more code. This excess can slow down web applications, leading to longer load times. For users, this can result in a poor experience, which is unacceptable in today’s digital environment.
There are several reasons why these performance issues arise:
- File Size: Libraries like Angular or React can be quite large, which may increase the amount of time taken for users to download and render web pages. This is especially critical for users with slower connections.
- Unoptimized Code: Many libraries come with heavy implementations. If developers are not careful, they may end up loading unnecessary functions or components which lead to excessive resources being utilized.
- Multiple Dependencies: Often, libraries depend on other libraries, creating chain reactions of loading. This can complicate the management of project dependencies, resulting in further performance drain.
To mitigate these issues, developers should critically analyze the libraries and frameworks they implement. Consider alternatives that might be leaner or investigate tree-shaking techniques in modern build processes, which help remove unused code.
Learning Curve for New Developers
The steep learning curve associated with many JavaScript libraries and frameworks can be daunting for new developers. While some frameworks, like Vue.js, aim for an easier learning experience, others, such as Angular, can overwhelm beginners with their complexity.
New developers might face the following challenges:
- Verbose Documentation: While detailed documentation is beneficial, it can also result in confusion if too extensive and not easily navigable. The abundance of information can discourage novices from even attempting to learn.
- Conceptual Overload: Frameworks often come with a variety of concepts to grasp, such as routing, state management, or component lifecycles. Beginners may struggle to see how these concepts interconnect.
- Community Resources: Although community support can be beneficial, the overwhelming amount of tutorials, articles, and forums can lead to information overload. Novices may have difficulty discerning which resources are reliable.
Encouragement from experienced developers is crucial in navigating these challenges. Effective mentorship can provide guidance and streamline the learning path for new developers, enhancing their competence with JavaScript libraries and frameworks.
Selecting a Suitable Library or Framework
Choosing the right JavaScript library or framework is crucial for the success of any web development project. Each option carries specific strengths and weaknesses, which can significantly influence the efficiency, flexibility, and maintainability of the application. Selecting a suitable library or framework requires a thoughtful consideration of several factors, including the nature of the project, team skills, and long-term goals. By aligning these elements thoughtfully, developers can enhance both productivity and quality of deliverables.
Assessing Project Requirements
When starting a new project, it is essential to first define the requirements clearly. What is the project's primary goal? Is it a single-page application or a complex data visualization tool? Both the scale and complexity should dictate the choice of library or framework.
Common aspects to evaluate include:
- Functionality Needed: Identify the specific features the application must support. For instance, if the focus is on real-time data, frameworks like React or Vue.js may be more suitable due to their efficient reactivity system.
- Performance Expectations: Some libraries optimize performance better than others. Assessing whether the application requires fast load times or can accommodate larger file sizes will guide the decision.
- Future Scalability: Consider whether the project might expand in scope later. A flexible framework that can adapt to growth will save effort in refactoring.
Clearly outlining requirements can avoid costly mistakes down the road, ensuring the selected library or framework fits well into the overall development strategy.
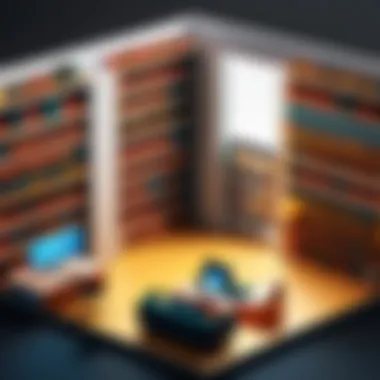
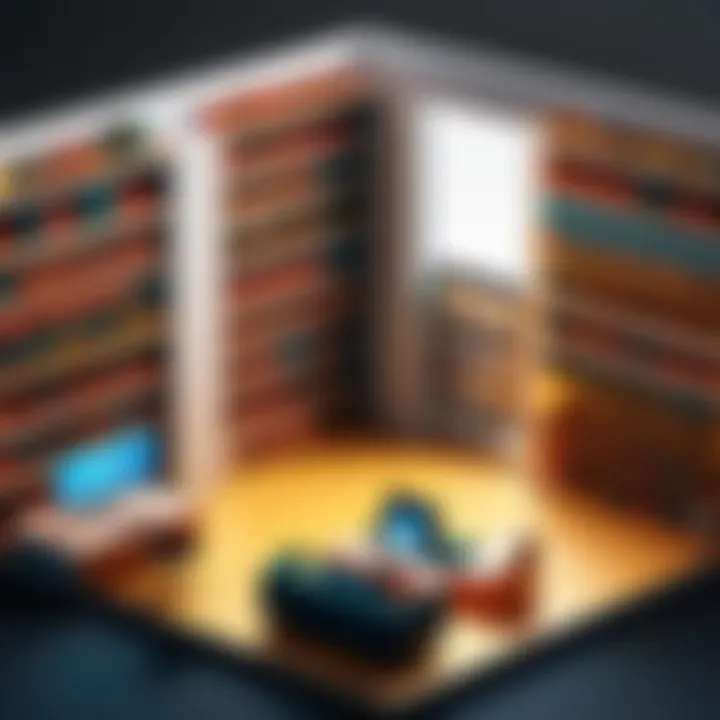
Aligning with Team Expertise
Once the project requirements are understood, the next step involves evaluating the team's existing skill sets. Using a library or framework that the team is already familiar with can significantly decrease the learning curve and accelerate development.
Consider the following points:
- Team Experience: Does the team have prior experience with a specific library or framework? Utilizing their strengths can lead to quicker problem-solving and smoother workflow.
- Learning Resources: If a team is eager to adopt a new tool, they should assess the availability of learning resources. Frameworks with rich documentation and community support, like Angular or React, will facilitate the learning process.
- Collaborative Environment: Teams also need to communicate effectively with one another. Adopting a library or framework that promotes collaboration helps share best practices, which enhances the overall development process.
Future Trends in JavaScript Libraries and Frameworks
The focus on future trends in JavaScript libraries and frameworks is essential for anyone immersed in the field of web development. As technology constantly evolves, keeping abreast of upcoming tools and practices can significantly affect the development process and end products. Emerging libraries and frameworks provide innovative functionalities while improving efficiency. Therefore, understanding these trends is not just beneficial but crucial.
Emergence of New Libraries and Frameworks
A key trend in the JavaScript ecosystem is the emergence of new libraries and frameworks. The demand for more specialized solutions is pushing developers to create unique tools that cater to specific needs. For instance, lightweight frameworks like Sapper and Svelte are gaining traction for their simplicity and performance efficiency. This trend allows developers to choose the right tool for their projects, which can streamline their workflow.
Moreover, modern libraries focus on features like reactivity and improved state management. Developers favor tools that minimize boilerplate code, facilitating faster deployments. Many new libraries also emphasize better integration with existing technologies and frameworks, such as GraphQL, which improves how data fetching is managed.
Here are some important points regarding the emergence of new libraries and frameworks:
- Increased focus on performance.
- Enhanced usability and simplicity.
- Specialized solutions aligned with current development needs.
Integration with Other Technologies
Integration with other technologies is another significant trend shaping the future of JavaScript libraries and frameworks. As applications become increasingly complex, the need to seamlessly integrate various technologies grows. This integration optimizes overall functionality and improves user experience. For example, libraries that connect directly with APIs or cloud services simplify data handling.
Frameworks like Next.js support server-side rendering in combination with React, enhancing performance and SEO capabilities. Additionally, the merging of JavaScript with TypeScript is gaining popularity. Many developers are adopting TypeScript for its type safety and error reduction, which ultimately results in better code quality.
The benefits of integration include:
- Enhanced compatibility with other programming languages and APIs.
- Improved performance through optimized interaction between frameworks and libraries.
- Greater scope for building complex applications efficiently.
Automatically adapting to new technologies allows developers to remain competitive. Monitoring these trends will empower developers to make informed choices and leverage tools that best fit their project requirements.
"In technology, as in all things, adaptability is critical to survival and success."
These insights into future trends will better prepare developers for the evolving landscape of JavaScript libraries and frameworks. Embracing change and continuously learning will ensure success in this dynamic environment.
Real-World Applications of JavaScript Libraries and Frameworks
JavaScript libraries and frameworks play a critical role in modern web and application development. Their real-world applications are vast, allowing developers to create efficient, scalable, and interactive solutions. By leveraging these tools, developers can benefit from ready-to-use functionality, which speeds up the development process significantly. Here, we will explore several key elements that highlight their importance.
Case Studies of Successful Implementations
Real-world applications of JavaScript libraries and frameworks can be seen in various successful projects across industries. For instance, Airbnb utilizes React for its front-end interface, enabling a seamless user experience. This choice allows developers to build interactive UIs efficiently and maintain them with ease.
Another example is the use of D3.js by the New York Times for data visualization articles. This library allows them to craft complex visual representations from data with relative simplicity. D3.js's ability to bind data to the Document Object Model (DOM) empowers journalists to tell stories through their data.
Moreover, e-commerce sites like Amazon leverage multiple frameworks and libraries to improve user engagement. By implementing various tools, they optimize product displays and enhance the overall customer journey. These implementations demonstrate the flexibility and strength of JavaScript libraries and frameworks in solving real-world challenges.
Evaluating Performance Metrics
When discussing the real-world applications of JavaScript libraries and frameworks, evaluating performance metrics becomes essential. Developers need to assess various aspects such as load times, rendering speed, and responsiveness.
For instance, a website using React may show decreased load times due to its virtual DOM implementation, which minimizes the updates made to the actual DOM. This decrease is crucial for maintaining user retention.
Additionally, tools like Google Lighthouse can be employed to measure performance impacts. These tools provide insights on various metrics including Time to Interactive (TTI) and First Contentful Paint (FCP). By continuously monitoring performance metrics, developers can make data-driven decisions regarding which libraries or frameworks to adopt.
Finale
In this article, we explored various aspects of JavaScript libraries and frameworks. These tools play a crucial role in modern web development, providing essential functionalities that streamline the development process. Understanding the distinctions between libraries and frameworks is necessary for making informed decisions on which to use.
Summarizing Key Takeaways
- Core Differences: Libraries provide reusable functions while frameworks offer a structured environment for development.
- Popular Choices: jQuery, D3.js, React, and Angular are among the widely used tools that have shaped the web landscape.
- Advantages: Increased efficiency, reusable code, and strong community support enhance project development.
- Challenges: Dependency management and learning curves can pose issues for developers.
By absorbing these key points, developers can better navigate the complexities associated with choosing the right tools for their projects.
Looking Ahead: The Evolution Continues
The realm of JavaScript is ever-evolving. With the rise of new libraries and frameworks, developers must stay adaptable. Trends like integration with artificial intelligence or enhancing performance will shape future developments. Emerging technologies will likely introduce innovative capabilities that could redefine how applications are built. As ever, being informed and prepared will be crucial for success in software development.
"Adapting to new trends and technologies is essential for maintaining relevance in the ever-changing landscape of web development."